1. 문제 번호 2480번
2. 문제 풀이
2.1 간단한 오름차순 방법(매우 효율적)
2.2 함수를 만들어서 중복데이터의 존재 여부 확인 (재귀함수??)
2.3 중복카운트(Stream)
나의 문제풀이 방식 및 순서
- 배열에 데이터를 넣어, if문으로 인덱스의 Value별로 비교하고 싶지 않아서 컬렉션을 사용 시도 (java스킬 향상 목적)
- HashMap 을 시도했지만 사용방법을 모름
- Stream 시도했지만 현재의 나로써 직관적이지 않음
- 이중 for문 시도
- > case1. input(6 6 6) 일때 인덱스 0,1,2 를 비교하였고 1,2를 비교하는 와중에 횟수가 더 증가해버림
input(6 3 6) 일때는 정상 작동 (return false를 사용하면 됐는데..)
3. 소스 인증
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 주사위 눈을 저장할 배열 생성
int[] dice = new int[3];
// 주사위 눈 입력 받기
for (int i = 0; i < 3; i++) {
dice[i] = scanner.nextInt();
}
// 주사위 눈을 오름차순으로 정렬
Arrays.sort(dice);
// 중복 여부 확인
if (dice[0] == dice[2]) {
// 같은 눈이 3개인 경우
System.out.println(10000 + dice[0] * 1000);
} else if (dice[0] == dice[1] || dice[1] == dice[2]) {
// 같은 눈이 2개인 경우
System.out.println(1000 + dice[1] * 100);
} else {
// 모두 다른 눈인 경우
System.out.println(dice[2] * 100);
}
}
}
import java.util.*;
import java.io.*;
class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine()," ");
List <Integer> dice = new ArrayList<>();
while(st.hasMoreTokens()){
dice.add(Integer.parseInt(st.nextToken()));
};
Map<Integer, Integer> countMap = new HashMap<>();
for(int d : dice) {
countMap.put(d, countMap.getOrDefault(d, 0) + 1);
}
int maxNum = Collections.max(dice);
switch(countMap.size()){
case 1:
System.out.print(10000+(maxNum*1000));
break;
case 2:
for(int key : countMap.keySet()) { //EntrySet() key Value 전체출력
if(countMap.get(key) == 2) {
maxNum = key;
break;
}
}
System.out.print(1000+(maxNum*100));
break;
default :
System.out.print(maxNum*100);
}
}
}
import java.util.*;
import java.lang.*;
import java.io.*;
/**********************
Writer : KTH
Purpose:
**********************/
class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine()," ");
List <Integer> dice = new ArrayList<>();
while(st.hasMoreTokens()){
dice.add(Integer.parseInt(st.nextToken()));
};
HashMap <Integer,Integer> mapCnt = dupliCnt(dice);
// 방법 1
// String[] names = null;
// names = new String[] { “혼공자”, “혼공족장”, “자바맨” };
// 방법 2
// String [] names = new String[3];
// int [] dice = new int [3];
// for(int i = 0; i<4; i++){
// dice.put(Integer.parseInt(st.nextToken()));
// };
// 1.dice안에 중복된 숫자의 갯수를 샌다.
// 2.중복횟수에 따른 수식을 나눈다.
// System.out.println(resultHour+" "+resultMinutes);
// HashMap에 중복된 숫자와 해당 숫자의 개수가 들어있는지 확인합니다.
for (Map.Entry<Integer, Integer> entry : mapCnt.entrySet()) {
System.out.println("중복된 숫자: " + entry.getKey() + ", 개수: " + entry.getValue());
}
}
public static HashMap<Integer,Integer> dupliCnt(List<Integer> dice){
HashMap<Integer, Integer> countMap = new HashMap<>();
for (int i = 0; i < dice.size(); i++) {
int num = dice.get(i);
countMap.put(num, countMap.getOrDefault(num, 0) + 1);
}
return countMap;
}
}
import java.util.*;
import java.lang.*;
import java.io.*;
// The main method must be in a class named "Main".
class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine()," ");
List<Integer> dice = new ArrayList<>();
while(st.hasMoreTokens()){
dice.add(Integer.parseInt(st.nextToken()));
}
Map<Integer,Integer> countMap = new HashMap<>();
for(int d : dice){
countMap.put(d, countMap.getOrDefault(d,0)+1);
}
//순차탐색
int maxNum = findMax(dice, 0, dice.size()-1);
switch(countMap.size()){
case 1:
System.out.print(10000+(maxNum*1000));
break;
case 2:
for(int key : countMap.keySet()) { //EntrySet() key Value 전체출력
if(countMap.get(key) == 2) {
maxNum = key;
break;
}
}
System.out.print(1000+(maxNum*100));
break;
default :
System.out.print(maxNum*100);
}
}
public static int findMax(List<Integer> data, int begin, int end){
if(begin==end){
return data.get(begin);
} else {
return Math.max(data.get(begin), findMax(data, begin+1, end));
}
}
}
4. 추가 개념
HaspMap getOrDefault
HashMap의 경우 동일 키 값을 추가할 경우 Value의 값이 덮어쓰기
String [] alphabet = { "A", "B", "C" ,"A"};
HashMap<String, Integer> hm = new HashMap<>();
for(String key : alphabet) hm.put(key, hm.getOrDefault(key, 0) + 1);
System.out.println("결과 : " + hm);
// 결과 : {A=2, B=1, C=1}
ArrayList
ArrayList vs 배열
ArrayList 특징
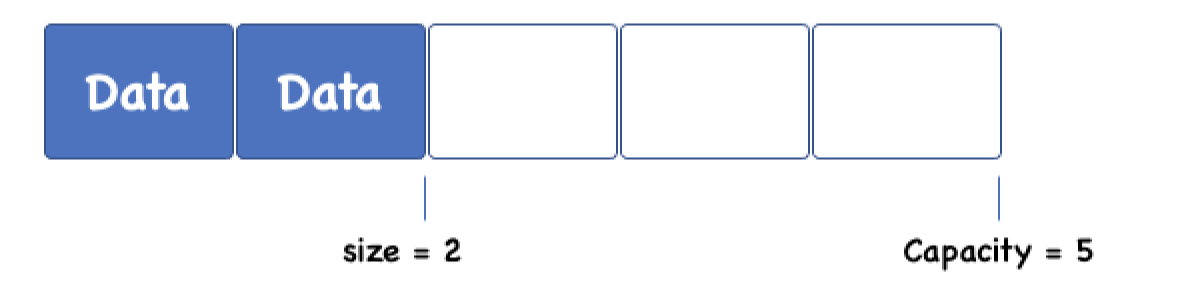
- 연속적인 데이터의 리스트 (데이터는 연속적으로 리스트에 들어있어야 하며 중간에 빈공간이 있으면 안된다)
- ArrayList 클래스는 내부적으로
Object[]배열을 이용하여 요소를 저장 - 배열을 이용하기 때문에 인덱스를 이용해 요소에 빠르게 접근할 수 있다.
- 크기가 고정되어있는 배열과 달리 데이터 적재량에 따라 가변적으로 공간을 늘리거나 줄인다.
- 그러나 배열 공간이 꽉 찰때 마다 배열을 copy하는 방식으로 늘리므로 이 과정에서 지연이 발생하게 된다.
- 데이터를 리스트 중간에 삽입/삭제 할 경우, 중간에 빈 공간이 생기지 않도록 요소들의 위치를 앞뒤로 자동으로 이동시키기 때문에 삽입/삭제 동작은 느리다.
- 따라서 조회를 많이 하는 경우에 사용하는 것이 좋다
배열 장단점
- 처음 선언한 배열의 크기(길이)는 변경할 수 없다. 이를 정적 할당(static allocation)이라고 한다.
- 데이터 크기가 정해져있을 경우 메모리 관리가 편하다.
- 메모리에 연속적으로 나열되어 할당하기 때문에 index를 통한 색인(접근)속도가 빠르다.
- index에 위치한 하나의 데이터(element)를 삭제하더라도 해당 index에는 빈공간으로 계속 남는다.
- 배열의 크기를 변경할 수 없기 때문에, 처음에 너무 큰 크기로 설정해주었을 경우 메모리 낭비가 될수 있고, 반대로 너무 작은 크기로 설정해주었을 경우 공간이 부족해지는 경우가 발생 할 수 있다.
// 타입설정 Integer 객체만 적재가능
ArrayList<Integer> members = new ArrayList<>();
// 초기 용량(capacity)지정
ArrayList<Integer> num3 = new ArrayList<>(10);
// 배열을 넣어 생성
ArrayList<Integer> list2 = new ArrayList<>(Arrays.asList(1,2,3));
// 다른 컬렉션으로부터 그대로 요소를 받아와 생성 (ArrayList를 인자로 받는 API를 사용하기 위해서 Collection 타입 변환이 필요할 때 많이 사용)
ArrayList<Integer> list3 = new ArrayList<>(list2);
출처: https://inpa.tistory.com/entry/JAVA-☕-ArrayList-구조-사용법#배열_장단점 [Inpa Dev 👨💻:티스토리]
🧱 자바 ArrayList 구조 & 사용법 정리
ArrayList 컬렉션 자바의 컬렉션 프레임워크를 접한다면 가장 먼저 배우는 컬렉션이 ArrayList 일 것이다. 자료구조(Data Structure) 이라고 해서 무언가 방대하게 느껴져 접근이 어려울 것 처럼 느끼겠지
inpa.tistory.com
'알고리즘(BOJ) 문제풀이' 카테고리의 다른 글
[BOJ/백준] 반복문_10950 (0) | 2024.05.08 |
---|---|
[BOJ/백준] 반복문_2739번 (0) | 2024.05.08 |
[BOJ/백준] 조건문_2884 (0) | 2024.05.07 |
[BOJ/백준] 조건문_2525 (0) | 2024.05.07 |
[BOJ/백준] 조건문_14681 (0) | 2024.05.03 |